Tutorial: Setup Single-SPA with Angular and React
Have you heard of Single-SPA? Simply put, it is a JavaScript metaframework that makes it possible to develop micro-frontends with different frameworks that can co-exist in a single application. In other words, with a Single-SPA (SPA stands for Single-Page-Application and yes, this means that the term Single-SPA contains an obvious redundancy), it is possible to split code according to functionality and, for example, to use Angular and React together in one application.
It is often rightly recommended not to use more than one framework for an application. However, there are situations where single-SPA is a good alternative, or where it is simply fun to use multiple frameworks.
Below is a short tutorial on setting up a Single-SPA with Angular and React. I wrote this tutorial myself using the script create-single-spa v.2.2.2. To follow this tutorial, set up your folder structure as follows:
my-first-single-spa
└─── angular-app
└─── react-app
└─── root-config
Step 1: Create Single-SPA root-config
Open a new terminal in the root directory of your project (in the folder my-first-single-spa) and run:
npx create-single-spa –moduleType root-config –layout
This will start a command line dialogue where you have to insert some data:
- directory to create root-config: root-config
- package manager: npm
- use TypeScript: no
- organization name: org1
That is all you need to create the root-config. If you now look in the root-config folder, you should see the files created.
Step 2: Create Angular app
Configuring the Single SPA to work seamlessly with Angular is quite a challenge. However, if you follow the steps below, it should work relatively well. Open a terminal in the root folder and enter:
npx create-single-spa --framework=angular
This will start a command line dialogue again, which you can answer with the following properties:
- directory to create angular app: angular-app
- project name: angular-mfe
- use Angular Routing: yes
- choose stylesheet format: css
- use Angular routing (yes this is redundant but required by the CLI): yes
- use BrowserAnimationsModule: yes
Now you need to do some configuration to connect Single-SPA and Angular. First, navigate to your newly created Angular application (here: cd angular-app/angular-mfe) and run npm install. Yes, this seems like a redundant step, but you need to do it as the create-single-spa-script does not do this.
After that, you need to configure Angular routing. Open app-routing.module.ts and add the following to the NgModule:
providers: [{ provide: APP_BASE_HREF, useValue: '/' }]
Then find the route array at the beginning of the file and add the following line to the array:
{ path: '**', component: EmptyRouteComponent }
Now go to app.module.ts and add EmptyRouteComponent to the declarations array in the NgModule.
Now go back to the root-config and register the Angular application. To do this, open root-config/src/index.ejs and remove the comment from the line that imports zone.js. In my version, this is line 61. If you don’t find this line right away, just search for zone or angular using CTRL+f.
Now look for the import map for registering applications in the index.ejs file. By default, this import map contains the Single-SPA welcome page, so you need to look for this line:
"@single-spa/welcome": "https://unpkg.com/single-spa-welcome/dist/single-spa-welcome.js"
Now register your Angular app by adding the following property to the import object:
"@org1/angular": "http://localhost:4200/main.js"
The final import map should look like this:
Now open the microfrontend-layout.html file located next to index.ejs and paste our Angular app inside the <route> tag:
<route default>
<application name="@org1/angular"></application>
</route>
Everything is already set up, so let’s see if everything works as expected. Open a new terminal and start the root configuration by typing this:
cd root-config
npm run start
Of course, you also have to start the Angular application. So start another terminal:
cd angular-app
npm run serve:single-spa:angular-mfe
Open your browser and go to http://localhost:9000. There you should see the Angular default template or anything you specified in the app component.
Step 3: Create React app
As with root-config and Angular-application, use the create-single-spa-script again.
npx create-single-spa --framework=react
And the following command line dialogue:
- directory to create angular app: react-app
- choose package manager: npm
- use TypeScript: yes
- organization name: org1
- project name: react
Of course, you also need to register the React app. So go to root-config/src/index.ejs, find the import map where you registered the Angular app in the previous step and add the following property to the import map:
"@org1/react": "http://localhost:8080/org1-react.js"
For the React app to work, you need to specify the React dependencies as common dependencies. To do this, you need to place another import map in the index.ejs. Since Single-SPA knows shared dependencies by default, you can simply look for a line that looks like this:
"single-spa": "https://cdn.jsdelivr.net/npm/single-spa@5.9.0/lib/system/single-spa.min.js",
After finding the line, add react and react-dom as common dependencies by naming these two properties after the Single-SPA dependency:
"react": "https://cdn.jsdelivr.net/npm/react@16.13.1/umd/react.production.min.js",
"react-dom": "https://cdn.jsdelivr.net/npm/react-dom@16.13.1/umd/react-dom.production.min.js"
Finally, you also need to make the React app known in the markup. Open microfrontend-layout.html and insert the React app as you did with the Angular app. The final result should look like this:
<route default>
<application name="@org1/angular"></application>
<application name="@org1/react"></application>
</route>
Start the React app by running npm run start in another terminal. Update localhost:9000 and from now on you should see an Angular app coexisting with a React app.
Did everything work as described? Do you have any comments or questions? Feel free to contact me or just leave a comment.
Notes:
Here you can finde more information about Single-SPA, FAQ, documents and also tutorials.
Mark Heimer has published two more articles in the t2informatik Blog:
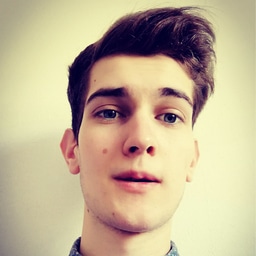
Mark Heimer
Mark Heimer works at t2informatik as a junior developer. He previously studied computer science at the Berlin School of Economics and Law. He writes about some of his experiences here in the blog.